Grunt is a task-based command line build tool for JavaScript that can be easily configured in Visual Studio 2015 to perform a variety of tasks such as minification or unit testing.
To use Grunt within our project we first need to make sure it is installed, this is done using NPM. To use NPM we need to create a configuration file (packages.json) in the root of our application. In the configuration file we can add the grunt dependency. We’ve also added a few other dependencies for other items we will use in this example.
// packages.json
{
"version": "1.0.0",
"name": "my application",
"devDependencies": {
"grunt": "~0.4.5",
"grunt-contrib-uglify": "~0.5.0",
"grunt-contrib-cssmin": "~0.14.0"
}
}
Adding the packages.json file to Visual Studio will automatically create a node_modules folder including all the devDependencies specified.
The devDependencies can specify exact versions or a range of versions for a dependency i.e.
- version must match version exactly
- >, <, >=, <= greater than, less than etc.
- ~version “Approximately equivalent to version”
- ^version “Compatible with version”
Now that grunt is installed locally for the project we can add a new Grunt Configuration file to the root of the project.
// gruntfile.js
module.exports = function(grunt) {
grunt.loadNpmTasks('grunt-contrib-uglify');
grunt.loadNpmTasks('grunt-contrib-cssmin');
grunt.initConfig({
pkg: grunt.file.readJSON('package.json'),
uglify: {
options: {
banner: '/*! <%= pkg.name %> <%= grunt.template.today("yyyy-mm-dd") %> */\n'
},
build: {
src: 'scripts/**/*.js',
dest: 'scripts/<%= pkg.name %>.min.js'
}
},
cssmin: {
target: {
files: [
{
expand: true,
cwd: 'css',
src: ['*.css', '!*.min.css'],
dest: 'css',
ext: '.min.css'
}
]
}
},
clean: ["build"]
});
grunt.registerTask('build', ['uglify', 'cssmin']);
}
In this grunt file, we are going to use the uglify plugin to minify and combine our js files in our script folder and then use cssmin to minify our css files in our css folder.
Now that grunt is installed and configured we can right click on the gruntfile.js file in our project and select Task Runner Explorer.
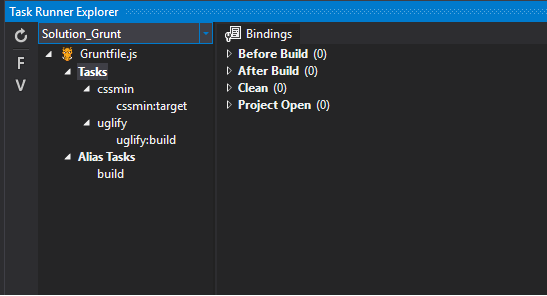
We can now either run the grunt tasks manually or bind them to events:
- Before build
- After build
- On clean
- On Project open
Git Ignore
If you are using source control it is recommended that the node_modules folder is not committed and that you allow NPM to resolve dependencies for the packages required i.e in git add the following to your .gitignore file
# =========================
# NPM ignore rules
# =========================
**/npm-debug.log
**/node_modules/*