ASP.NET WebHooks is a new lightweight sub/pub model for wiring together Web Api and SaaS services.
On the receiving side, it provides a common method for subscribing and receiving web hooks from SaaS services. Out the box, it comes with support for Azure Alerts, Dropbox, GitHub, MailChimp, And many other SaaS services.
On the sending side, it provides a model for managing subscribers and the ability to notify them about events.
Receiving WebHook Notifications
ASP.NET WebHooks are enabled by installing a NuGet package for the provider or providers you want to receive WebHooks for. The packages are named Microsoft.AspNet.WebHooks.Receivers.{{ServiceName}
. In this example, we will use the GitHub WebHooks package Microsoft.AspNet.WebHooks.Receivers.GitHub
GitHub WebHooks
WebHooks is a pattern that varies service to service, but the basic idea is the same. Event notifications in a service are propagated as an HTTP POST request containing information about the event itself.
For GitHub, the WebHook HTTP POST request contains a JSON object including information about the event.
{
"ref": "refs/heads/master",
"before": "7d447c66b69ff208d2cf6a5ce5c1fa4810c19025",
"after": "4343646f26609ca8296be0d1ddb8ca94f917b6fa",
...,
"commits": [
{
"id": "4343646f26609ca8296be0d1ddb8ca94f917b6fa",
"distinct": true,
"message": "Changed content of text file ...",
...
}],
...
}
In the case of the GitHub receiver a X-Hub-Signature
HTTP header with a hash of the request body is also included that is checked and verified by the receiver implementation.
Basic WebHook flow:
- WebHook sender exposes events that a client can subscribe to
- WebHook receiver subscribes by registering, giving a URL for where the event notification should be posted to and a secret key that is used to sign the HTTP POST request body
- When an event happens the sender finds all matching registrations and makes HTTP POST requests to the registered URLs
GitHub WebHook Configuration
Once the Nuget Package has been installed two configuration values need to be setup in GitHub. By installing ASP.NET WebHooks you get a WebHook controller that accepts WebHook requests from services. By default the URI of this controller is:
- https://{{Host}}/api/webhooks/incoming/{{receiver}}
This is the URI that you should set the GitHub Payload URL to.
The second configuration value is the secret key, this should be set to an unpredictable value such as an SHA256 hash. The secret key should then be stored in an AppSetting called MS_WebHookReceiverSecret_GitHub
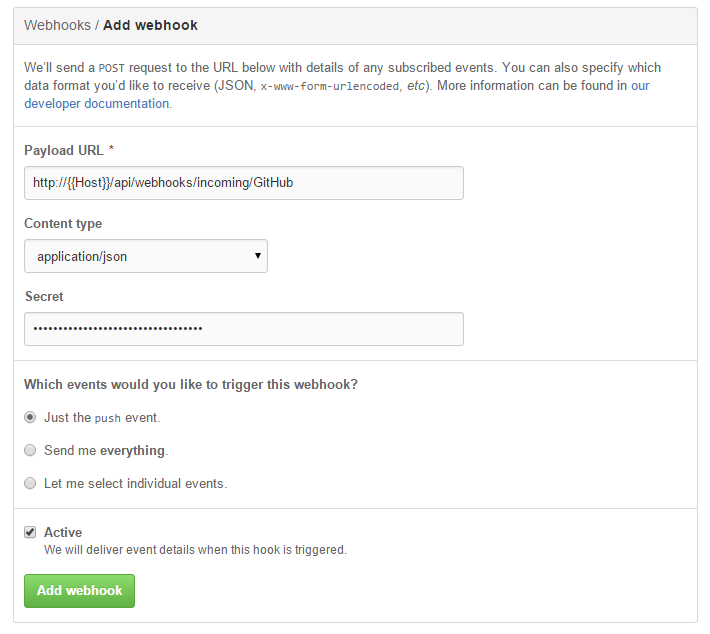
Going back to the code, two things need to be done. We first need to configure the receiver in our application. This is done by calling an extension method on the HttpConfiguration object typically within the WebApiConfig class.
public static class WebApiConfig
{
public static void Register(HttpConfiguration config)
{
// Web API configuration, services & routes
...
// Load GitHub receiver
config.InitializeReceiveGitHubWebHooks();
}
}
When a WebHook event is received one or more handlers are called so next we need to create a GitHubHandler
extending the WebHookHandler
class to run any custom logic required.
public class GitHubHandler : WebHookHandler
{
public override Task ExecuteAsync(string receiver, WebHookHandlerContext context)
{
if (receiver.Equals("GitHub", StringComparison.InvariantCultureIgnoreCase))
{
string action = context.Actions.First();
JObject data = context.GetDataOrDefault<JObject>();
}
return Task.FromResult(true);
}
}
Result
When deployed adding a new commit means the handler will receive an event notification.
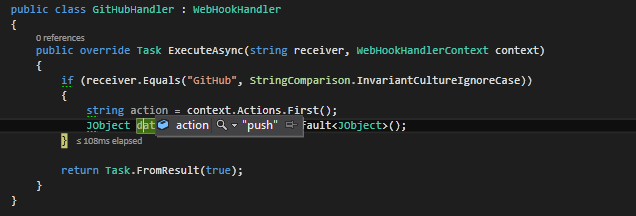